C Programming (10+2), Important Programs with source codes ( Part III, Strings)
- ΔIO
- Nov 15, 2022
- 2 min read
Updated: Nov 21, 2022
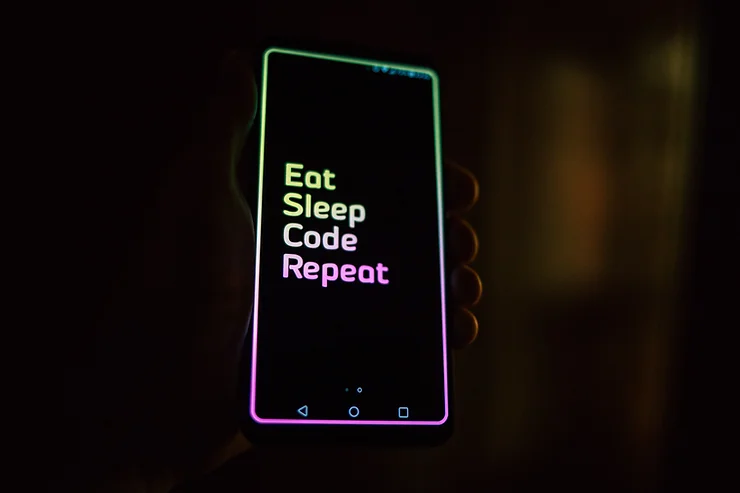
Strings in C
Following is the list of string functions and their usage that we will be using in C programming.
String Function | Usage |
strlen() | Prints length of string |
strcat() | Concatenate or Joins two or more strings |
strcmp() | Compares two strings (e.g. equal or not) |
strcpy() | Copies string |
strrev() | Reverse string (string - gnirts) |
strlwr() | Converts string into lowercase letters |
strupr() | Converts string into uppercase letters |
Don't forget to include string library before doing main function. [ #include <string.h> ]
Let's see examples of each string function.
strlen() , strupr() , strlwr()
Program to take a string as input and convert it into lowercase, and uppercase and print its length.
#include<stdio.h>
#include<conio.h>
#include<string.h>
void main()
{
char st[20];
int len;
char low[20],upr[20];
printf("Enter any string:");
scanf("%s",st);
// length of string
len = strlen(st);
printf("Lowercase of string = %s\n",strlwr(st));//lowercase
printf("Uppercase of string = %s\n",strupr(st));//uppercase
printf("Length of string = %d",len);
}
strcpy()
Program to copy one string into another.
#include<stdio.h>
#include<conio.h>
#include<string.h>
void main()
{
char st1[]="Computer";
char st2[10];
strcpy(st2,st1);
puts(st2);
}
P.S syntax of strcpy() is strcpy(destination_string, source_string);
strrev()
Program to reverse a string "Computer".
#include<stdio.h>
#include<conio.h>
#include<string.h>
void main()
{
char name[8] ="Computer",name2[8];
strcpy(name2,name);
strrev(name);
printf("The reversed string is %s",name);
}
strcmp()
Program to take two strings as input and find out which one is greater.
#include<stdio.h>
#include<conio.h>
#include<string.h>
void main()
{
char st1[20],st2[20];
printf("Enter first string: ");
scanf("%s",st1);
printf("Enter second string: ");
scanf("%s",st2);
if (strcmp(st1,st2)>0)
{
printf("Greater is %s",st1);
}
else
printf("Greater string is %s",st2);
}
Syntax for strcmp() is strcmp(string1,string2);
Most Important Questions for Exam
Program to input a word and determine whether it is a palindrome or not. [ Palindrome: Same spell from front and back ( eye )]
#include <stdio.h>
#include <string.h>
void main()
{
char word[30],word1[30];
printf("Enter a string: ");
scanf("%s",word);
strcpy(word1,word);
if (strcmp(word1,strrev(word))== 0)
{
printf("The string is palindrome.");
}
else
{
printf("The string is not palindrome.");
}
}
Program to input the names of ‘n’ numbers of students and sort them in alphabetic order. [Most Important: Generally asked in 10 marks]
#include<string.h>
#include<conio.h>
#include<string.h>
void main()
{
int n,i,j;
char name[30][25],temp[25];
//max. 30 names
printf("Enter the number of students: ");
scanf("%d",&n);
for(i=0;i<n;i++)
{
printf("Enter the name of %d student :",n-i);
scanf("%s",name[i]);
}
for(i=0;i<n;i++)
{
for(j=i+1;j<n;j++)
{
if(strcmp(name[i],name[j])>0)
{
strcpy(temp,name[i]);
strcpy(name[i],name[j]);
strcpy(name[j],temp);
}
}
}
printf("\nSorted List of students:\n");
for(i=0;i<n;i++)
{
printf("%d. %s \n",i,name[i]);
}
}
Extra Dose !!
Program to read the age of 10 students and count the number of students the aged between 15 and 22.
void main()
{
int age[40],i,c=0;
for(i=0;i<10;i++)
{
printf("Enter the age of %d students : ",10-i);
scanf("%d",&age[i]);
}
for(i=0;i<40;i++)
{
if(age[i]>=15 && age[i]<=22)
c++;
}
printf("No. of students of age between 15 to 22 = %d",c);
}
All the programs on this site are checked and are totally integrated, If you encounter any problem you can submit your report here.
Comments