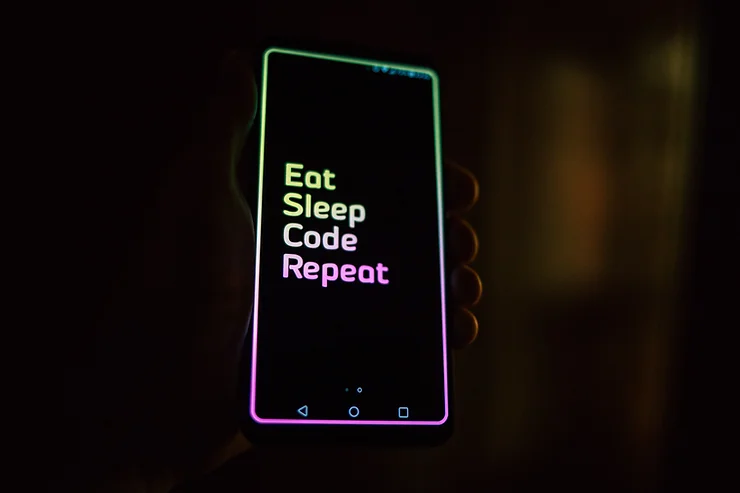
STRUCTURE
A structure is a collection of variables under a single name. The variable may be of different types and each has a name that is used to select it from the structure. The keyword ‘struct’ is used to declare the structure variable.
Some features of the structure are as follows :
1. It can be treated as a record that is a collection of interrelated data fields with
data fields having different data types.
2. It allows a nested structure that is one structure inside another
structure.
3. Passing structure variables as parameters to any
function is possible.
4. It is possible to copy one structure variable to another by
simple assignment operator(=).
SYNTAX :
struct structure_name
{
data_type member_variable1;
data_type member_variable2;
.....
};
EXAMPLE :
struct student
{
int rn;
char name[30];
char address[35];
char phone[20];
}std[20];
Important Programs based on Structure
Program to input name, roll, and reg-number of 10 students using structure and display in the proper format.
#include<stdio.h>
void main()
{
struct student
{
char name[35];
int roll,reg;
}s[5];
int i;
for(i=0;i<10;i++)
{
printf("Enter your name, roll, reg of SN %d.\n",i+1);
printf("Name : ");
scanf("%s",&s[i].name);
printf("Roll : ");
scanf("%d",&s[i].roll);
printf("Reg_No : ");
scanf("%d",&s[i].reg);
printf("\n");
}
printf("Printing the structure records:");
for(i=0;i<5;i++)
{
printf("\nName : %s",s[i].name);
printf("\nRoll : %d",s[i].roll);
printf("\nReg : %d",s[i].reg);
}
}
Program to input the five employee names and salaries using the structure and display the records in the proper format.
#include <stdio.h>
void main()
{
struct employee
{
char name[25];
int salary;
}e[5];
int i;
for(i=0;i<5;i++)
{
printf("\nEnter the name of the employee no %d :",i+1);
scanf("%s",&e[i].name);
printf("Enter the salary of the employee no %d :",i+1);
scanf("%d",&e[i].salary);
}
printf("\nPrinting the information of the employees");
for(i=0;i<5;i++)
{
printf("\n The name of the employee no %i is :%s",i+1,e[i].name);
printf("\n The salary of the employee no %i is :%d\n\n",i+1,e[i].salary);
}
}
Union
Difference between Structure and Union.
Structure | Union |
Each member of a structure is assigned its own unique storage. It takes more memory space than union. | All members of a union share the same storage area of computer memory. It takes less memory than that of structure. |
All the members of the structure can be accessed at any point in time. | Only one member of a union can be accessed at any given time. |
The structure can be declared as: struct student { char name[20]; int roll; float marks; }st; | The union can be declared as: union student { char name[20]; int roll; float marks; }st; |
Difference between structure and array.
Structure | Array |
A structure can have elements of different types. | A structure cannot have elements of different types. |
A structure is a user defined data type. | An array is a derived data type. |
First, we have to define a structure and then declare the variable of that type. | An array behaves like a built-in data type. We can declare an array variable and use it. |
SYNTAX struct structure_name { data_typemember_variable1; data_typemember_variable2; ..... }; | SYNTAX datatype array name[size]; |
EXAMPLE struct student { int rn; char name[30]; char address[35]; char phone[20]; }std[20]; | 5. EXAMPLE { int a[5],i; printf(“enter five numbers”); for(i=0;i<5;i++) { scanf(“%d”,&a[i]); } for(i=0;i<5;i++) { printf(“\n %d”,a[i]); } |
All the programs on this site are checked and are totally integrated, If you encounter any problem you can submit your report here.
Browse All Topics on C Programming
Comments