When it comes to data visualization and computer vision, Python is the best-used programming of all. Python provides libraries like Open CV and Mediapipe which are pretty easy to use for computer vision. In this tutorial, we will develop a program using Python that can detect hands as shown in the picture below.
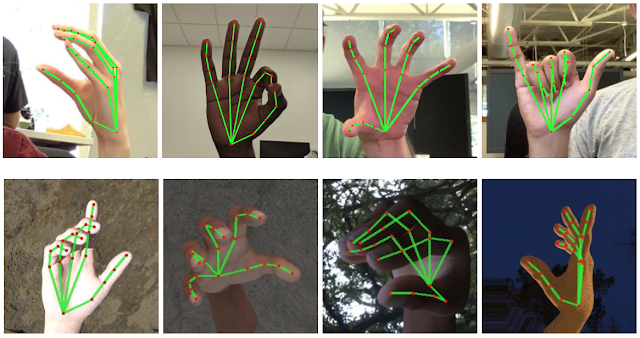
Let's unpack libraries.
Open CV
OpenCV is a library of programming functions mainly aimed at real-time computer vision. It was originally developed by Intel and later supported by Willow Garage then Itseez. The library is cross-platform and free for use under the open-source Apache 2 License.
Mediapipe
Mediapipe is a cross-platform library developed by Google that provides amazing ready-to-use ML solutions for computer vision tasks.
Source Code
import cv2
import mediapipe as mp
import time
cap = cv2.VideoCapture(0)
mpHands = mp.solutions.hands
hands = mpHands.Hands()
mpDraw = mp.solutions.drawing_utils
pTime = 0
cTime = 0
while True:
success, img = cap.read()
imgRBG = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
results = hands.process(imgRBG)
print(results.multi_hand_landmarks)
if results.multi_hand_landmarks:
for handLms in results.multi_hand_landmarks:
for id, lm in enumerate(handLms.landmark):
# print(id,lm)
h, w, c = img.shape
cx, cy = (int(lm.x * w), int(lm.y * h))
print(id, cx, cy)
if id == 0:
cv2.circle(img, (cx,cy), 15, (255,0,255), cv2.FILLED)
mpDraw.draw_landmarks(img, handLms, mpHands.HAND_CONNECTIONS)
cTime = time.time()
fps = 1/(cTime-pTime)
pTime = cTime
cv2.putText(img,str(int(fps)),(10,70),cv2.FONT_HERSHEY_PLAIN,3,(255,0,255),3)
cv2.imshow("Image",img)
cv2.waitKey(1)
You can view and get the source code in GitHub from here.
Comments